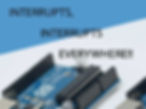
OVERVIEW
Using Interrupts enables you to run some code only when a change happens on a Pin.
Without interrupts your Arduino would spend most of it’s time just monitoring Pins for activity and slow down the rest of your code.
But most Arduino boards have a limited number of available Interrupt Pins.
The UNO for example only has 2 of them: Interrupt 0 on Pin 2 and Interrupt 1 on Pin 3.
Thankfully, using a simple library, you can make any Pin on your Arduino an Interrupt Pin, so in theory you could have as many of them as you have available Pins.
In this tutorial, we will use a Rotary Encoder which will control some LED’s when turned on way or another.
We will use the standard Interrupt 0 on Pin 2, but also with the help of the Library, create another Interrupt on Pin 11 to detect when we use the Rotary Encoder.
PARTS USED
WS2812 RGB Stick
Arduino UNO
Rotary Encoder Module
These are Amazon affiliate links...
They don't cost you anything and it helps me keep the lights on
if you buy something on Amazon. Thank you!
CONNECTIONS

The Rotary Encoder PinCLK pin will be connected to Pin 2 (Interrupt 0) ORÂ Pin 11 to test the created Interrupt.
*PinCLK is either connected to Pin 2 OR Pin 11, not both at the same time…
PinDT is connected to Pin 4
Voltage and Ground Pin are connected to 3.3V and Ground on the UNO
The RGB LED stick DI (Digital In) pin is connected to Pin 5
Voltage and Ground Pin are connected to 5V and Ground on the UNO
THE CODE
To create Interrupts on any pin we are using the ‘PinChange Interrupt’ Library.
We are also using the ‘FastLed’ Library to control the RGB LED stick.
You can look at other tutorials we have done in the past for more information on how to use this library.
As you can see in the code, we created 2 Interrupt function that basically do the same thing but are connected to different Pins: Interrupt 0 (Pin 2) and Interrupt 11 (Pin 11) that was created by the Library.
As always you can have a look at the tutorial video for more information.
/* Start of Code */
#include "PinChangeInt.h" // PinChange Interrupt library
#include "FastLED.h" // FastLED library
// WS2812 RGB Stick connection
#define led_pin 5 // RGB Stick DI pin connected to UNO pin 5
#define NUM_LEDS 8 // # of WS2812 LEDs on stick
CRGB leds[NUM_LEDS]; // FastLED Library Init
// Rotary Encoder Module connections
#define PinCLK 2 // Rotary CLK pin connected to UNO interrupt 0 on pin 2
#define PinDT 4 // Rotary DT pin connected to UNO pin 4
#define PinCLK_pin11 11 // Rotary CLK pin connected to UNO normal pin 11
volatile boolean led_changed; // to detect that led need to be changed
volatile int led_to_light=0; // number of led to light up
// Interrupt routine on Pin 2 (Interrupt zero)runs if CLK pin changes state
void rotarydetect_pin2 () {
delay(1); // delay for Debouncing Rotary Encoder
if (digitalRead(PinCLK)) {
if (digitalRead(PinDT)) {
if (led_to_light > 1){
led_to_light=led_to_light-1; }}
if (!digitalRead(PinDT)) {
if (led_to_light < 9){ led_to_light=led_to_light+1; }} led_changed = true; } } // Interrupt routine on Pin 11 runs if CLK pin changes state void rotarydetect_pin11 () { delay(1); // delay for Debouncing Rotary Encoder if (digitalRead(PinCLK_pin11)) { if (digitalRead(PinDT)) { if (led_to_light > 1){
led_to_light=led_to_light-1; }}
if (!digitalRead(PinDT)) {
if (led_to_light < 9){
led_to_light=led_to_light+1; }}
led_changed = true;
}
}
void setup () {
FastLED.addLeds<NEOPIXEL,led_pin>(leds, NUM_LEDS); // Setup FastLED Library
FastLED.clear();
FastLED.show();
pinMode(PinCLK,INPUT); // Set Pin 2 (Interrupt zero) to Input
pinMode(PinCLK_pin11, INPUT); // Set normal 8 pin to Input
pinMode(PinDT,INPUT);
attachInterrupt (0, rotarydetect_pin2, CHANGE); // interrupt 0 always connected to pin 2 on Arduino UNO
attachPinChangeInterrupt(PinCLK_pin11, rotarydetect_pin11, CHANGE); // interrupt connected to pin 11
}
void loop () {
// Runs if Rotary Encoder rotation is detected
if (led_changed) {
led_changed = false; // do Not repeat IF loop until new rotation detected
// Which LED's to light up
FastLED.clear();
for(int x = 0; x != (led_to_light - 1); x++) {
if (x < 2) leds[x] = CRGB::Red; if (x > 1 & x < 5) leds[x] = CRGB::Orange; if (x > 4) leds[x] = CRGB::Green; }
FastLED.setBrightness(50);
FastLED.show();
}
}
/* End of Code */
TUTORIAL VIDEO
DOWNLOAD
Copy the above Sketch code in your Arduino IDE software to program your Arduino.
You can download the PinChange Interrupt Library here…