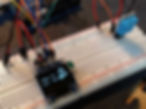
OVERVIEW
OLED Displays are great in many ways.
They use very little power, are bright, easy to read with large viewing angle and have high resolution considering their small size.
The OLED we will use today is .96″ inch in size, features 128×64 pixels and uses the SPI Bus.
We will use the u8glib library to communicate with our display.
This library has many available parameters, we will use some of them to display a bitmap image and to display some text.
If you would like to experiment and learn more about this library, you can go to the Wiki: U8GLIB Wiki.
In this tutorial we will use the DHT11 Temperature and Humidity sensor and display those value on the OLED Display.
PARTS USED
128x64 SPI OLED Display
Arduino UNO
DHT-11 Temperature Sensor
These are Amazon affiliate links...
They don't cost you anything and it helps me keep the lights on
if you buy something on Amazon. Thank you!
CONNECTIONS

Our OLED display uses the SPI Bus.
Since the SPI Bus is faster than I2C, this will make our display very responsive.
Of course there’s a tradeoff, the SPI Bus uses more pins on our UNO (5 compared to 2).
But for our project this is not a problem.
The OLED is connected to pins 9 through 13 and the DHT11 is connected to pin A0.
We used a breadboard to connect the VCC and Ground to both modules from our UNO.
THE CODE
We are using 2 libraries in this tutorial: u8glib for the OLED display and the dhtlib for the DHT11 sensor.
We also used a little program to convert our bitmap image into hexadecimal to display it on the display.
Again, please watch our tutorial video for more information.
#include "U8glib.h"
#include "dht.h"
#define dht_apin A0
dht DHT;
U8GLIB_SH1106_128X64 u8g(13, 11, 10, 9, 8); // D0=13, D1=11, CS=10, DC=9, Reset=8
const uint8_t brainy_bitmap[] PROGMEM = {
0x00, 0x00, 0x03, 0xB0, 0x00, 0x00, 0x00, 0x00, 0x07, 0xFC, 0x00, 0x00, 0x00, 0x00, 0x0C, 0x46,
0x00, 0x00, 0x00, 0x00, 0xFC, 0x47, 0xC0, 0x00, 0x00, 0x01, 0xCE, 0x4C, 0x60, 0x00, 0x00, 0x03,
0x02, 0x58, 0x30, 0x00, 0x00, 0x03, 0x02, 0x58, 0x10, 0x00, 0x00, 0x02, 0x02, 0x58, 0x18, 0x00,
0x00, 0x03, 0x06, 0x4C, 0x18, 0x00, 0x00, 0x07, 0x04, 0x44, 0x18, 0x00, 0x00, 0x0D, 0x80, 0x40,
0x3C, 0x00, 0x00, 0x09, 0xC0, 0x40, 0xE6, 0x00, 0x00, 0x18, 0x78, 0x47, 0xC2, 0x00, 0x00, 0x18,
0x0C, 0x4E, 0x02, 0x00, 0x00, 0x1F, 0x86, 0x4C, 0x7E, 0x00, 0x00, 0x0E, 0xC6, 0xE8, 0xEE, 0x00,
0x00, 0x18, 0x43, 0xF8, 0x82, 0x00, 0x00, 0x10, 0x06, 0x4C, 0x03, 0x00, 0x00, 0x30, 0x0C, 0x46,
0x01, 0x00, 0x00, 0x30, 0x18, 0x46, 0x01, 0x00, 0x00, 0x10, 0x18, 0x43, 0x03, 0x00, 0x00, 0x18,
0x10, 0x43, 0x03, 0x00, 0x00, 0x1C, 0x70, 0x41, 0x86, 0x00, 0x00, 0x0F, 0xE0, 0x40, 0xFE, 0x00,
0x00, 0x09, 0x1E, 0x4F, 0x06, 0x00, 0x00, 0x08, 0x30, 0x43, 0x86, 0x00, 0x00, 0x0C, 0x20, 0x41,
0x86, 0x00, 0x00, 0x06, 0x60, 0x40, 0x8C, 0x00, 0x00, 0x07, 0x60, 0x40, 0xB8, 0x00, 0x00, 0x01,
0xE0, 0x41, 0xF0, 0x00, 0x00, 0x00, 0x38, 0xE3, 0x00, 0x00, 0x00, 0x00, 0x0F, 0xBE, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x1F, 0xCF, 0x82, 0x0C, 0x86, 0x46, 0x1F, 0xEF, 0xC3, 0x0C,
0xC6, 0xEE, 0x1C, 0xEC, 0xC7, 0x0C, 0xE6, 0x7C, 0x1C, 0xED, 0x8D, 0x8C, 0xFE, 0x38, 0x1C, 0xED,
0x8D, 0xCC, 0xDE, 0x38, 0x1D, 0xCD, 0xDF, 0xCC, 0xCE, 0x38, 0x1F, 0x8C, 0xF8, 0xEC, 0xC6, 0x38,
0x1F, 0xEC, 0x08, 0x0C, 0xC2, 0x18, 0x1C, 0xEC, 0x00, 0xC0, 0x00, 0x00, 0x1C, 0xFD, 0xFB, 0xC0,
0x00, 0x00, 0x1C, 0xFC, 0x63, 0x00, 0x00, 0x00, 0x1C, 0xEC, 0x63, 0xC0, 0x00, 0x00, 0x1F, 0xEC,
0x60, 0xC0, 0x00, 0x00, 0x1F, 0xCC, 0x63, 0xC0, 0x00, 0x00, 0x1F, 0x0C, 0x63, 0x00, 0x00, 0x00,
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x28, 0x2B, 0x4F, 0x67,
0x42, 0x38, 0x7B, 0xEA, 0x86, 0xB2, 0x28, 0xC7,
};
void draw(void) {
u8g.drawBitmapP( 76, 5, 6, 50, brainy_bitmap); // put bitmap
u8g.setFont(u8g_font_unifont); // select font
u8g.drawStr(0, 30, "Temp: "); // put string of display at position X, Y
u8g.drawStr(0, 50, "Hum: ");
u8g.setPrintPos(44, 30); // set position
u8g.print(DHT.temperature, 0); // display temperature from DHT11
u8g.drawStr(60, 30, "c ");
u8g.setPrintPos(44, 50);
u8g.print(DHT.humidity, 0); // display humidity from DHT11
u8g.drawStr(60, 50, "% ");
}
void setup(void) {
}
void loop(void) {
DHT.read11(dht_apin); // Read apin on DHT11
u8g.firstPage();
do {
draw();
} while( u8g.nextPage() );
delay(5000); // Delay of 5sec before accessing DHT11 (min - 2sec)
}
TUTORIAL VIDEO
DOWNLOAD
Copy and paste the above code in the Arduino IDE to program your Arduino.
Used Libraries:
Download LCDAssistant software:
Download the U8GLib library here:
Download the DHT library here: https://github.com/RobTillaart/Arduino/tree/master/libraries/DHTlib