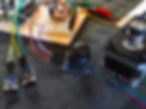
OVERVIEW
Since we made the tutorial on how to control a stepper motor using an analog joystick, we’ve gotten a lot of request on how to have the stepper move when moving the joystick and not move back to the center.
So in this tutorial we will do just that, but also add some limit switches and add the ability to adjust the speed.
The limit switches will be used to limit the travel of the stepper.
To control the speed we will use the switch included on the joystick module to set the speed.
PARTS USED
EasyDriver Stepper Driver
Joystick Module
Stepper Motor NEMA 17
These are Amazon affiliate links...
They don't cost you anything and it helps me keep the lights on
if you buy something on Amazon. Thank you!
CONNECTIONS

Here are the connections:
Pin 9 is connected to Steps pin on Easy Driver Pin 8 is connected to Direction pin Pin 10 is connected to MS1 pin Pin 11 is connected to MS2 pin Pin 12 is connected to SLEEP pin
Pin A0 connected to joystick X-axis Pin 4 is connected to the joystick Key or switch pin
Pint 2 and 3 are connected to the OUT pin of the Limit Switches
The Ground and Voltage pins of the Easy Driver are connected to a 1 Amp 12V power supply.
We are also using a small breadboard to split the Ground and Voltage connections of the various devices
THE CODE
The code we are using does not require any libraries, but you could use one to control the Stepper motor to give you more options if you want.
By checking the analog value of the X-axis of the joystick we know which direction to move the stepper motor. 512 is center, zero is full left and 1023 is full right.
So in our code we check if the analog value is higher than 712 to detect a right movement and a value lower than 312 will indicate a move to the left.
We than check the condition of the limit switches, and if there are not activated, we proceed to move the motor.
Using the Switch Case function, we change the delay to control the speed when we press down on the joystick switch.
As always, please check out the tutorial video to have more information.
#define step_pin 9 // Pin 9 connected to Steps pin on EasyDriver
#define dir_pin 8 // Pin 8 connected to Direction pin
#define MS1 10 // Pin 10 connected to MS1 pin
#define MS2 11 // Pin 11 connected to MS2 pin
#define SLEEP 12 // Pin 12 connected to SLEEP pin
#define X_pin A0 // Pin A0 connected to joystick x axis
#define Joy_switch 4 // Pin 4 connected to joystick switch
#define Limit01 2 // Pin 2 connected to Limit switch out
#define Limit02 3 // Pin 3 connected to Limit switch out
int step_speed = 10; // Speed of Stepper motor (higher = slower)
void setup() {
pinMode(MS1, OUTPUT);
pinMode(MS2, OUTPUT);
pinMode(dir_pin, OUTPUT);
pinMode(step_pin, OUTPUT);
pinMode(SLEEP, OUTPUT);
pinMode(Limit01, INPUT);
pinMode(Limit01, INPUT);
pinMode(Joy_switch, INPUT_PULLUP);
digitalWrite(SLEEP, HIGH); // Wake up EasyDriver
delay(5); // Wait for EasyDriver wake up
/* Configure type of Steps on EasyDriver:
// MS1 MS2
//
// LOW LOW = Full Step //
// HIGH LOW = Half Step //
// LOW HIGH = A quarter of Step //
// HIGH HIGH = An eighth of Step //
*/
digitalWrite(MS1, LOW); // Configures to Full Steps
digitalWrite(MS2, LOW); // Configures to Full Steps
}
void loop() {
if (!digitalRead(Joy_switch)) { // If Joystick switch is clicked
delay(500); // delay for deboucing
switch (step_speed) { // check current value of step_speed and change it
case 1:
step_speed=10; // slow speed
break;
case 3:
step_speed=1; // fast speed
break;
case 10:
step_speed=3; // medium speed
break;
}
}
if (analogRead(X_pin) > 712) { // If joystick is moved Left
if (!digitalRead(Limit01)) {} // check if limit switch is activated
else { // if limit switch is not activated, move motor clockwise
digitalWrite(dir_pin, LOW); // (HIGH = anti-clockwise / LOW = clockwise)
digitalWrite(step_pin, HIGH);
delay(step_speed);
digitalWrite(step_pin, LOW);
delay(step_speed);
}
}
if (analogRead(X_pin) < 312) { // If joystick is moved right
if (!digitalRead(Limit02)) {} // check if limit switch is activated
else { // if limit switch is not activated, move motor counter clockwise
digitalWrite(dir_pin, HIGH); // (HIGH = anti-clockwise / LOW = clockwise)
digitalWrite(step_pin, HIGH);
delay(step_speed);
digitalWrite(step_pin, LOW);
delay(step_speed);
}
}
}
TUTORIAL VIDEO
DOWNLOAD
Copy the above Sketch code in your Arduino IDE software to program your Arduino.